关于Java IO流的学习笔记
一、File类
1.1 FIle类的理解
- (1)java.io.File类:文件和文件目录路径的抽象表达形式,与平台无关
- (2)File功能:新建、删除、重命名文件和目录。但不能打开文件,访问内容
- (3)在Java程序中表示文件/目录,必须要有一个File对象。但是File对象,不一定存在真实的本地文件/目录
- (4)File对象可以作为参数传递给流的构造器
1.2 FIle类的实例化(构造器)
1 | (1)File file = new File(String pathName);---文件路径作为参数 |
1 | 举例: |
1.3 File类的常用方法
1 | public String getAbsolutePath():获取绝对路径 |
1 | 只适用于文件目录: |
1 | public boolean rename(File dest):把文件重命名为指定的文件路径(即:把文件进行剪切并且重命名) |
1 | 判断条件: |
1 | 硬盘中创建文件 / 文件目录 |
1 | 硬盘中删除文件: |
1.4 总结
- File类中涉及到关于文件后文件目录的创建、删除、重命名、修改时间、文件大小等方法,并未涉及到写入或读取文件内容的操作。如果需要读取或写入文件内容,必须使用IO流来完成
- 后续File类的对象常会作为参数传递到六的构造器中,指定读取或写入的“终点”
二、IO流
2.1 流的分类
- 按操作数据单位不同:字节流(8 bit)、字符流(16 bit)
- 按数据流的流向不同:输入流、输出流
- 按流的角色不同:节点流、处理流
2.2 四个流的抽象基类
抽象基类 | 字节流 | 字符流 |
---|---|---|
输入流 | InputStream | Reader |
输出流 | OutputStream | Writer |
- 后面的流都是这些了流的派生
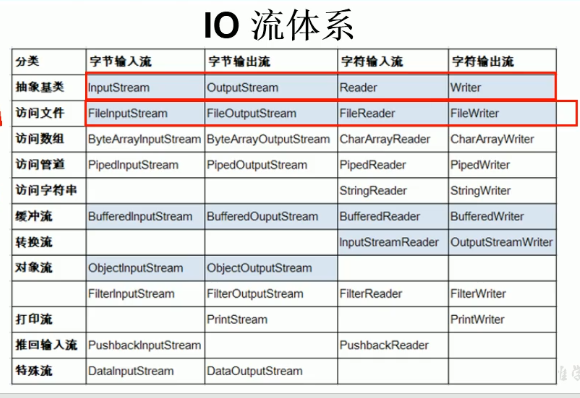
三、文件流(常用流1)
3.1 文件流种类
文件流 | 字节流 | 字符流 |
---|---|---|
输入流 | FileInputStream | FileReader |
输出流 | FileOutputStream | FileWriter |
- 就在抽象基类中多加了个”File”
3.2 FileReader使用
- 操作步骤:
- 1.指明文件对象
- 2.提供具体的流
- 3.数据的读入
- 4.流的关闭操作
- 5.try-catch-finally处理异常,finally填写资源的关闭操作
1 | FileReader fr = null;//5.需要异常处理 |
- 写入数据的两种方法:
- (1)调用空参方法
read()
,返回读取的整数数据,需要强转转为字符
- (1)调用空参方法
1 | int data; |
- (2)创建一个字符数组,调用
read(char[] arr)
方法,将数据读取数组里,返回一个读取长度
- (2)创建一个字符数组,调用
1 | char[] cbuf = new char[5];//数组长度随意 |
3.3 FileWriter使用
- 操作步骤:
- 1.指明文件对象
- 2.提供具体的流,参数2是boolean型,true是继续文件内容往下写,false是覆盖文件内容重新写
- 3.数据的写入
- 4.流的关闭操作
- 5.try-catch-finally处理异常,finally填写资源的关闭操作
1 | 此处省略了第五步:异常处理 |
- 读取数据的两种方法:
- (1)调用
write(String str)
,进行数据写入
- (1)调用
1 | fw.write("I have a dream!\n"); |
- (2)调用
write(char[] arr, int start, int end)
,传入一个字符数组,写入这数组从arr[start],到arr[end]的数据,一般配合FileReader的read(char[])来使用
- (2)调用
1 | char[] cbuf = new byte[1024]; |
3.4 FileInputStream 和 FileOutputStream使用
- 用法与字符流的FileReader 和 FileWriter区别不大
- 读取的数据变成字节,所以创建数组存储时,应该使用byte[]
- 字节流适合用于非文本文件,例如:(.jpg, .mp3, .mp4, .ppt, .doc …)
四、缓冲流(常用流2)
4.1 缓冲流分类
缓冲流 | 字节流 | 字符流 |
---|---|---|
输入流 | BufferedInputStream | BufferedReader |
输出流 | BufferedOutputStream | BufferedWriter |
4.2 缓冲流说明
- 缓冲流作用:提高流的读取,写入速度
- 提高读写速度的原因:内部提供了一个缓冲区
- 缓冲流属于处理流的一种,处理流就是“套接”在已有的流的基础上
4.3 使用方法
- 在原来的流,套一个缓冲流。即创建一个缓冲流,用原来的流作为参数
1 | 以:文件的复制为例 |
4.4 额外说明
- 缓冲流的用法和文件流的用法几乎没有差异
- 缓冲流的BufferedReader,提供了一个新的方法
String readline()
,可以读取一整行的数据,但该数据不包括换行符,需要自己添加换行符
五、转换流(常用流3)
5.1 转换流分类
转换流 | 流 | 作用 |
---|---|---|
输入流 | InputStreamReader | InputStream –> Reader(解码) |
输出流 | OutPutStreamWriter | Writer –> OutputStream(编码) |
5.2 转换流说明
- 作用:提供了字节流和字符流之间的转换
- 转换流也是处理流的一种,套在原有流的基础上
5.3 使用方法
- 方法和缓冲流一样,要套在原有的流,使字节流变成字符流,字符流变成字节流
1 | 例:对文件进行重新编码 |
5.4 额外补充
- 创建转换流会有个参数2,参数2填写的是字符集
- 字符集种类:
- ASCII:美国标准信息交换码
- ISO8859-1:拉丁码表。欧洲码表
- GB2312:中国的中文编码表,最多两个字节编码所有字符
- GBK:中国的中文编码表升级,融合了更多的中文文字符号,最多两个字节编码
- Unicode:国际标准码,融合了目前人类使用的所有字符
- UTF-8:目前最常用的字符集,编程的编码方式,可用1-4个字节表示一个字符
六、其他流(不常用)(了解)
6.1 标准输入 / 输出流
- 种类:
System.in
:标准的输出流(字节流),默认从键盘输入System.out
:标准输出流(字节流),默认从控制台输出
- 说明:
- 标准流本身就是一个流,不需要创建对象,可以直接使用
- System类的
setIn(InputStream is)
/setOut(PrintStream os)
,重新指定输入和输出的流
1 | 使用例子: |
6.2 打印流
- 种类:
- PrintStream
- PrintWriter
- 说明:
- 打印流,将基本数据类型的数据格式转化为字符串输出
- 操作:
- (1)创建一个输出流
- (2)
PrintStream ps = new PrintStream(输出流, autoFlush:true/false)
//创建一个打印流 - (3)
System.setOut(打印流)
:修改了System.out的打印位置,从控制台打印,变成到输出流所在位置 - (4)调用
System.out.print()
和System.out.println()
来使用打印流
6.3 数据流
- 种类:
- DataInputStream
- DataOutputStream
- 说明:
- 数据流:用于读写或写出基本数据类型的变量或字符串
- 用数据流写入的文件,也只能用数据流读取,其他方式读取会出现乱码。并且读取的顺序,要按照写入顺序就行读取
1 | 例:演示写入以及读取 |
七、对象流
7.1 定义
- 作用:用于存储和读取基本数据类型数据 或 对象的处理流
- 方法:
- ObjectInputStream:(序列化)保存基本数据类型数据或对象的机制
- ObjectOutputStream:(反序列化)读取基本数据类型或对象的机制
7.2 使用方法
- 序列化
1 |
|
- 反序列化
1 |
|
7.3 自定义类序列化要求
- (1)实现Serializable (标识接口,不需要重写任何方法)
- (2)提供全局常量,标识UID
public static final long serivalVersionUID = 1231231L;
- (3)除了当前Person类需要实现Serializeable,其类中属性,除了基本数据类型,其余属性也要实现Serializable接口
八、RandomAccessFile
8.1 定义
- 1.RandomAccessFile直接继承与java.lang.Object类,实现了DataInput和DataOutput接口
- 2.RandomAccessFile即可以作为一个输入流,又可以作为一个输出流
8.2 构造器
public RandomAccessFile(File file, String mode)
public RandomAccessFile(String name, String mode)
mode参数:
- r:以只读方式打开
- rw:打开以便读取和写入
- rwd:打开以便读取和写入;同步文件内容的更新
- rws:打开以便读取和写入;同步文件内容和元数据的更新
8.3 使用方法
- 使用的方法和 FileInputStream 和 FileOutputStream区别不大
- 如果RandomAccessFile作为输出流,写出到的文件如果不存在,则在执行过程中自动创建,如果写出到文件存在,则对原有文件内容进行覆盖
- RandomAccessFile类,额外新增的方法
public void seek(int pos)
//将指针调到角标为pos的位置,可通过此方法实现从文件末尾添加
1 | 通过seek()方法,来实现插入效果 |