通过Java获取数据库连接,学习笔记
一、导入驱动jar包
注意:本文使用的是IDEA集成编辑软件,mysql8.0数据库
(1)”File” —> “Project Structure”
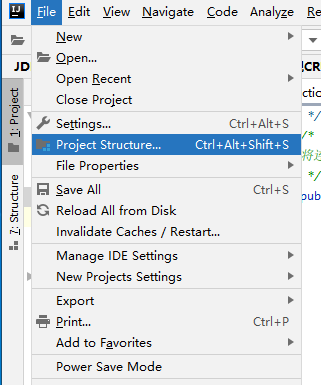
- (2)”Model” —> “Dependeincies” —> “+” —> “JARs or directories”
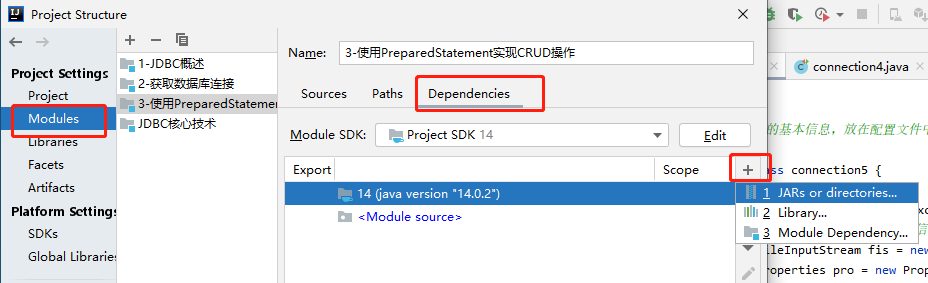
- (3)选择jar包所在路径,然后”OK”即可
二、获取数据库连接
- 以下会列出5种数据库连接方法,但每个方法是上一个方法的迭代,第五个版本是最终版,也是最推荐连接的方法
2.1 连接方式一
1 |
|
- 说明:
- (1)连接数据的方法:
Connection connect(String url, java.util.Properties info)
- (2)url格式:
jdbc:mysql://localhost:3306/test?serverTimezone=GMT%2B8
jdbc:mysql
– 协议localhost
– ip地址3306
– 端口号test
– test数据库?serverTimezone=GMT%2B8
– 解决时区问题
- (1)连接数据的方法:
2.2 连接方式二
- 方式二是对方式一的优化,因为方式一出现了第三方的API
Driver driver = new com.mysql.cj.jdbc.Driver();
- (上面代码出现了
com.sql.cj.jdbc.Driver()
第三方API)
- (上面代码出现了
- 可以通过反射来加载类,来创建对象
1 |
|
2.3 连接方式三
- 使用DriverManager来代替Driver来获取连接
- DriverManger连接数据库的方式,要比Driver简单,且方式多
- 使用DriverManager要先进行注册驱动,才能获取连接
- 注册驱动:
public static void registerDriver(java.sql.Driver driver)
- 连接数据库:
public static Connection getConnection(String url)
public static Connection getConnection(String url, java.util.Properties info)
public static Connection getConnection(String url, String user, String password)
- 常用第三种方法连接数据库
- 注册驱动:
1 |
|
2.4 连接方式四
- 是方式三的优化,省略了方式三的一些不必要的操作
- 反射获取驱动类会自动进行DriverManager的驱动注册,可以进行省略
1 |
|
2.5 连接方式五(最终版)
- 使用配置文件来读取连接数据库所需要的信息
- 程序进行封装之后,可以直接修改配置文件而改变要读取的内容,而不用重新打开编辑器进行修改
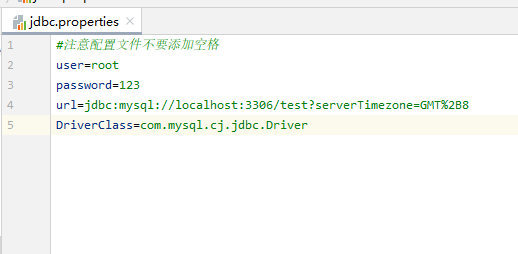
1 |
|