关于Java如何操作Excel的读写
1 Excel处理
1.1 相关技术
- Apache POI
- easyExcel
2 POI
2.1 介绍
- POI提供API给Java程序对Micorsoft Office格式档案读和写的功能
2.2 基本功能
- HSSF – 提供读写 Microsoft Excel 格式方案的功能(03版excel)
- XSSF – 提供读写Microsoft Excel OOXML格式档案的功能(07版excel)
- HWPF – 提供读写Microsoft Word 格式档案的功能
- HSLF – 提供读写Microsoft PowerPoint 格式档案的功能
- HDGF – 提供读写Microsoft Visio 格式档案的功能
2.3 Excel内容划分
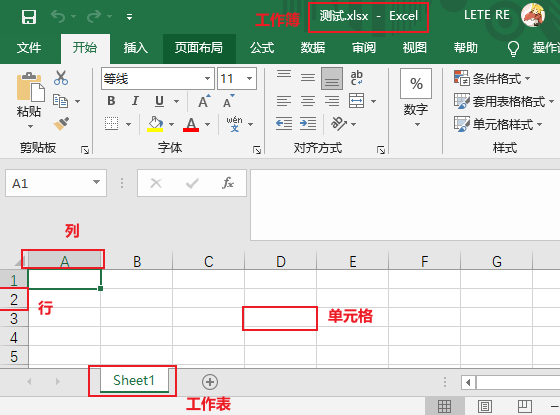
2.4 Excel写入
- (1)依赖导入
1
2
3
4
5
6
7
8
9
10
11
12
13<!-- apache-poi:处理office文件(03版excel-xls) -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>5.0.0</version>
</dependency>
<!-- apache-poi (07版excel-xlsx) -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>5.0.0</version>
</dependency>
- (2)写入代码
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
public void wirte03Test() throws Exception{
//1、创建03版工作簿
Workbook workbook = new HSSFWorkbook();
//2、创建工作表
Sheet sheet = workbook.createSheet("测试表");
//3、创建单元格
Row row0 = sheet.createRow(0); //行
Cell cell = row0.createCell(0);//列
//第一行第一列,对应excel的(1, A)单元格
//4、写入数据
cell.setCellValue("Up主名单");
//5、将工作簿存储到磁盘上
FileOutputStream fos = new FileOutputStream("./src/main/resources/excel/03Excel.xls"); //创建流
workbook.write(fos); //写入磁盘
fos.close(); //关闭流
}
//03与07基本一致,创建对象该为XSSFWorkBook,存储文件后缀名改为xlsx
2.5 大数据写入
- (03版)HSSF
- 优点:过程中写入缓存,不操作磁盘,最后一次性写入磁盘,速度快
- 缺点:最多处理65536行,否则会抛出异常
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
public void writeBig03Test() throws Exception {
//1、创建工作簿
Workbook workbook = new HSSFWorkbook();
//2、创建工作表
Sheet sheet = workbook.createSheet("测试表");
//3、循环写入数据
for(int rowNum = 0; rowNum < 65536 ; rowNum ++) {
Row row = sheet.createRow(rowNum);
for (int cellNum = 0 ; cellNum < 5 ; cellNum++) {
Cell cell = row.createCell(cellNum);
cell.setCellValue("( " + rowNum + ", " + cellNum + " )");
}
}
//4、写入磁盘
FileOutputStream fos = new FileOutputStream("./src/main/resources/excel/writeBig03.xls");
workbook.write(fos);
fos.close();
}
- (07版)XSSF
- 优点:处理的数据更多
- 缺点:写数据非常慢,非常消耗内存,会发生内存溢出
- (07版升级)SXSSF
- 优点:可以写非常大的数据量,写数据的速度更快,占用更少内存
- 缺点:过程会产生临时文件,需要清理临时文件(默认100条记录保存在内存,超过会将前面数据写入临时文件,可以通过带参创建对象时,带定义内存保存数据个数)
- 通过调用其
dispose()
方法来处理临时文件,子类特有方法
2.6 Excel读取
1 |
|
2.7 判断读取数据类型
1 |
|
3 EasyExcel
3.1 介绍
- EasyExcel是阿里巴巴开源的一个excel处理框架,以使用简单、节省内存著称
- EasyExcel能大大减少占用内存的主要原因在解析Excel时没有讲文件数据一次性全部加载到内存中,而是从磁盘上一行行读取数据,逐个解析
3.2 简单写入
- (1)依赖引入
1
2
3
4
5
6<!-- alibaba-easyExcel:excel处理 -->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>easyexcel</artifactId>
<version>2.2.8</version>
</dependency>
- (2)创建实体类
1
2
3
4
5
6
7
8
9
10
11
public class Person {
//表标题
private String name;
private String sex;
//忽略字段
private Integer age;
}
- (3)写入数据
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
public void simpleWrite() {
//1、创建数据(List集合)
List<Person> data = new ArrayList<>();
Person person1 = new Person();
person1.setName("C酱");
person1.setSex("女");
data.add(person1);
//2、写入数据
String fileName = "./src/main/resources/excel/person.xls";
EasyExcel.write(fileName, Person.class) //文件路径,写入对象
.sheet(0, "表1") //工作表号,表名
.doWrite(data); //写入数据
}
2.3 高级写入
2.4 简单读取
- (1)创建监听器
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15public class PersonListener extends AnalysisEventListener<Person> {
//easyExcel会一条一条数据进行读取
//读取一条数据调用方法
public void invoke(Person person, AnalysisContext analysisContext) {
System.out.println(person); //打印读取到的数据
}
//读取完所有数据调用方法
public void doAfterAllAnalysed(AnalysisContext analysisContext) {
System.out.println("已全部读取完毕!");
}
}
- (2)读取数据
1
2
3
4
5
6
7
8
public void simpleRead() {
//1、读取数据
String fileName = "./src/main/resources/excel/person.xls";
EasyExcel.read(fileName, Person.class, new PersonListener()) //文件路径 对象 监听器
.sheet(0) //工作表
.doRead(); //读取
}
2.5 高级读取
- (1)读取多表数据
1 | public void repeatedRead() { |
2.4 小结
- EasyExcel并没有比较详细的讲解,因为EasyExcel是中国人所写的,有中文文档,读官方文档压力不大,也更加详细,推荐读取官方文档