关于Java高频面试题学习
1 JavaSE
1.1 自增变量
1 |
|
1.2 单例模式
- 饿汉式:直接创建对象,不存在线程安全问题(3种)
1 | public class Singleton1 { |
1 | public enum Singleton2 { |
1 | public class Singleton3 { |
- 懒汉式:延迟创建对象(3种)
1 | public class Singleton4 { |
1 | public class Singleton5 { |
1 | public class Singleton6 { |
1.3 类初始化和实例化过程
- (1)类初始化过程
- main函数所在类先初始化
- 子类初始化需要先初始化父类
- 按代码顺序执行静态类变量、静态代码块
- (2)类实例化过程
- 子类实例化需要先实例化父类
- 按代码顺序执行非静态变量、非静态代码块
- 构造器永远最后执行
- (3)重写
- 子类重写父类方法,父类调用方法时是调用子类的重写方法
1 | // 父类 |
1 | /* |
1.4 传参机制
- 基本数据类型:传递值
- 引用数据类型:传递地址值
- String和包装类:无法修改值,改变值需要新创空间,重新指向新空间
1 | public class ParamTest { |
1.5 递归和迭代
- 递归:
- 优点:大问题转化为小问题,可以减少代码量,同时代码精简,可读性好
- 缺点:递归调用浪费了空间,而且递归太深容易造成堆栈溢出
- 迭代:
- 优点:代码运行效率好,因为时间只因循环次数增加而增加,而且没有额外的空间开销
- 缺点:代码不如递归简洁,可读性差
1 | /* |
1 | /** |
1 | /** |
1.6 局部变量和成员变量
- 弄清楚变量作用域即可
1 | public class VariableTest { |
2 SSM
2.1 SpringBean作用域
1 | <!-- scope属性用于指定bean的作用域 --> |
- Spring的Bean默认作用域为singleton,即单例,只会实例化一个
- 其他的作用域:
类别 | 说明 |
---|---|
singleton | 在SpringIOC容器中仅存在一个 Bean实例,spring启动自动创建 |
prototype | 每次调用getBean()都会返回一个新的实例 |
request | 每次http请求 都会创建一个新Bean,仅适用于WebApplicationContext环境 |
session | 同一个http session共享一个Bean ,不同http session使用不同的Bean,仅适用于WebApplicationContext环境 |
2.2 事务传播属性
- 事务传播行为:开启事务的A方法运行在另一个开启事务的B方法,事务运行的是A的,还是B的
1 |
|
- 事务传播属性通过
@Transactional(propagation=Propagation.XXXX)
来设置 - 以下事务参数都添加在A方法
参数 | 说明 |
---|---|
REQUIRED(默认) | 使用B方法的事务 |
REQUIRES_NEW | 使用A方法的事务 |
SUPPORTS | |
NOT_SUPPORTED | |
MANDATORY | |
NEVER | A方法无法运行在有事务的B方法,报异常 |
NESTED |
2.3 事务隔离级别
- 事务并发引出问题
问题 | 说明 |
---|---|
脏读 | 读取到别的事务更新但未提交 的数据 |
不可重复读 | 事务A读取数据后,事务B修改并提交数据,事务A重新读取数据发现不一致 |
幻读 | 事务A读取表的数据,事务B向表插入新行,事务A重新读取表行数变多了 |
- 隔离级别
- 可通过
@Transactional(isolation=Isolation.XXXXX)
来设置方法的隔离级别
参数 | 说明 |
---|---|
READ_UNCOMMITTED (读未提交) | 允许读取修改但未提交的数据 |
READ_COMMITTED (读已提交) | 只能读取已提交数据(避免脏读) |
REPEATABLE_READ (可重复读) | 对某字段进行操作时,其他事务无法操作此字段 (避免不可重复读) |
SERIALIZABLE (串行化) | 对某个表进行操作时,其他事务无法操作此表数据(避免幻读) |
2.4 POST/GET请求中文乱码
- POST:配置过滤器
1
2
3
4
5
6
7
8
9
10
11
12
13
14<filter>
<filter-name>CharacterEncodingFilter</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<!-- 参数初始化 -->
<init-param>
<param-name>encoding</param-name>
<param-value>utf-8</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>CharacterEncodingFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
- GET:修改tomcat的server.xml文件(tomcat8及以上版本不用配置)

2.5 SpringMVC工作流程
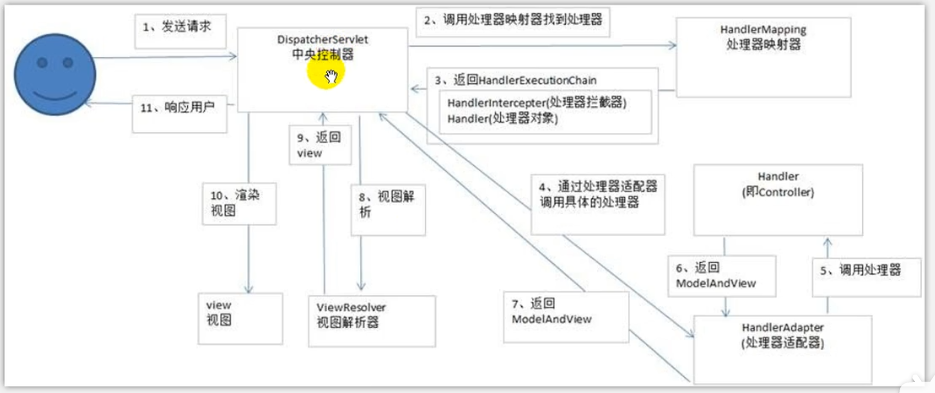
2.6 Mybatis驼峰命名
- (1)sql语句起别名
1
2
3
4select
last_name lastName
from
table
- (2)配置开启驼峰命名
1
2
3<settings>
<setting name="mapUnderscoreToCamelCase" value="true"/>
</settings>
- (3)创建resultMap自定义映射
1
2
3
4<resultMap id="MyEmp" type="Dao.Employee">
<id column="id" property="id"/>
<result column="last_name" property="lastName"/>
</resultMap>
3 Java高级
3.1 linux服务类指令
3.2 git分支命令与应用
- 命令
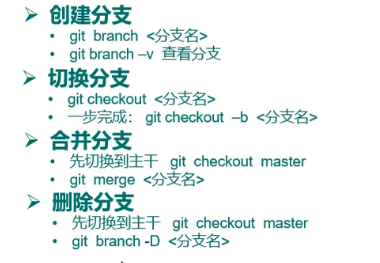
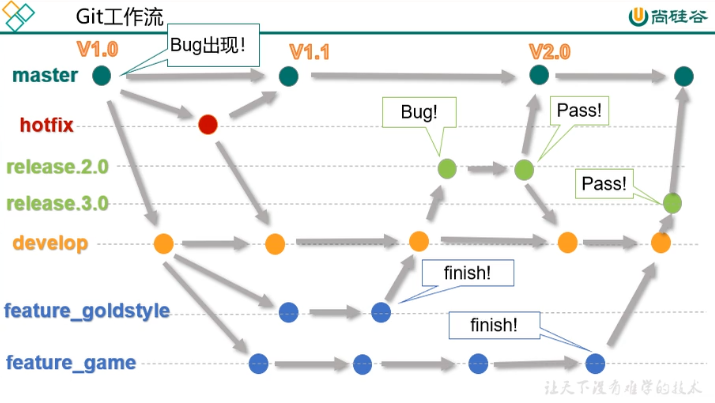
3.3 redis持久化
3.4 mysql索引
- 适合建立索引情况
- (1)主键自动建立唯一索引
- (2)频繁查询字段应建立索引
- (3)外键建立索引