EL表达式说明和使用方式
1 EL表达式介绍
- EL:Expression Language(表达式语言)
- 作用:简化并替换JSP页面的表达式脚本,用来在JSP页面输出数据
- 示例
1
2
3
4
5<body>
<% request.setAttribute("key", "value"); %>
JSP表达式:<%= request.getAttribute("key") %> <br/>
EL表达式:${key}
</body>
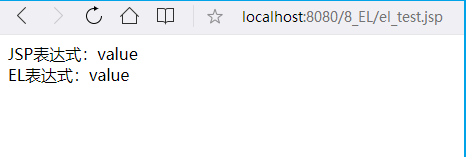
2 EL表达式作用域
- EL表达式主要用来输出四大域对象中的数据
- 四大域对象存储相同key名的数据,优先输出范围最小的域对象的数据
1 | <body> |
3 常用数据结构取值
- 实例
1 | // 创建一个类, 里面包含平时常见的数据结构 |
1 | <body> |
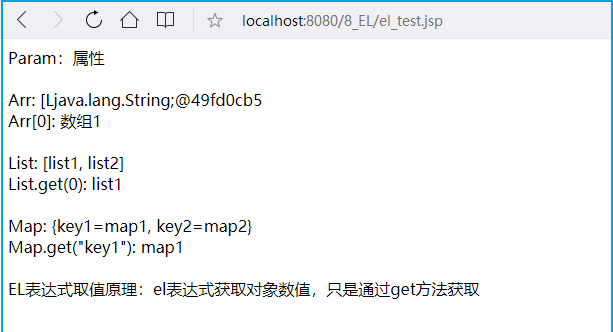
4 运算
4.1 关系运算
关系运算符 | 说明 | 示例 | 结果 |
---|---|---|---|
== 或 eq | 等于 | ${5 == 5} |
true 或 false |
!= 或 ne | 不等于 | ${5 != 5} |
true 或 false |
< 或 lt | 小于 | ${5 < 5} |
true 或 false |
<= 或 le | 小于等于 | ${5 <= 5} |
true 或 false |
> 或 gt | 大于 | ${5 > 5} |
true 或 false |
>= 或 ge | 大于等于 | ${5 >= 5} |
true 或 false |
4.2 逻辑运算
逻辑运算符 | 说明 | 示例 | 结果 |
---|---|---|---|
&& 或 and | 与运算 | ${5 == 5 && 11 == 11} |
true 或 false |
ll 或 or | 或运算 | ${5 == 5 ll 11 == 11} |
true 或 false |
! 或 not | 取反运算 | ${!true} |
true 或 false |
4.3 算术运算
算术运算符 | 说明 | 示例 | 结果 |
---|---|---|---|
+ | 加法 | ${ 1 + 1 } |
|
- | 减法 | ${ 1 - 1 } |
|
* | 乘法 | ${ 1 * 1 } |
|
/ 或 div | 除法 | ${ 1 / 1 } |
|
% 或 mod | 取模 | ${ 1 % 1 } |
4.4 empty运算
- empty运算:判断一个数据是否为空,为空则true,反之false
- 语法:
${ empty 数据 }
- 数据为空的情况
- 值为null
- 空字符串
- 数组长度为0,且类型为Object
- list集合长度为0
- map集合长度为0
4.5 三元运算
- 语法:
${ 表达式1 ? 表达式2 : 表达式3 }
- 表达式1为true,执行表达式2,反之执行表达式3
5 11个隐含对象
- EL表达式中,自定义了11个隐含对象,可以直接拿来使用
变量名 | 类型 | 作用 |
---|---|---|
pageContext | PageContextImpl |
获取Jsp中九大内置对象 |
pageScope | Map<String, Object> |
获取pageContext域中的数据 |
requestScope | Map<String, Object> |
获取requestContext域中的数据 |
sessionScope | Map<String, Object> |
获取session域中的数据 |
applicationScope | Map<String, Object> |
获取application域中的数据 |
param | Map<String, String> |
获取request中的请求参数值 |
paramValues | Map<String, String[]> |
获取request中的请求参数值,存在多个值时使用 |
header | Map<String, String> |
获取request中请求头参数值 |
headerValues | Map<String, String[]> |
获取request中的请求参数值,存在多个值时使用 |
cookies | Map<String, Cookie> |
获取当前请求中的cookie信息 |
initParam | Map<String, String> |
获取在web.xml中<context-param> 的参数值 |