JSTL标签库学习记录
1 介绍
1.1 基础介绍
- JSTL:JSP Standard Tag Library(JSP标准标签库)
- 主要替代JSP的代码脚本部分,使代码更加简洁
- 五种标签库
功能范围 URI 前缀 核心标签库(重点) http://java.sun.com/jsp/jstl/core c 格式化 http://java.sun.com/jsp/jstl/fmt fmt 函数 http://java.sun.com/jsp/jstl/functions fn 数据库(不使用) http://java.sun.com/jsp/jstl/sql sql XML(不使用) http://java.sun.com/jsp/jstl/xml x
1.2 使用
- (1)引入依赖(jar)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28<!-- tomcat10以下 -->
<!-- https://mvnrepository.com/artifact/org.apache.taglibs/taglibs-standard-impl -->
<dependency>
<groupId>org.apache.taglibs</groupId>
<artifactId>taglibs-standard-impl</artifactId>
<version>1.2.5</version>
<scope>runtime</scope>
</dependency>
<!-- https://mvnrepository.com/artifact/org.apache.taglibs/taglibs-standard-spec -->
<dependency>
<groupId>org.apache.taglibs</groupId>
<artifactId>taglibs-standard-spec</artifactId>
<version>1.2.5</version>
</dependency>
<!--tomcat10-->
<!-- https://mvnrepository.com/artifact/org.glassfish.web/jakarta.servlet.jsp.jstl -->
<dependency>
<groupId>org.glassfish.web</groupId>
<artifactId>jakarta.servlet.jsp.jstl</artifactId>
<version>2.0.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/jakarta.servlet.jsp.jstl/jakarta.servlet.jsp.jstl-api -->
<dependency>
<groupId>jakarta.servlet.jsp.jstl</groupId>
<artifactId>jakarta.servlet.jsp.jstl-api</artifactId>
<version>2.0.0</version>
</dependency>
- (2)JSP引入标签库
- 脚本:
<%@ taglib prefix="前缀" uri="URI地址" %>
2 Core标签库
2.1 <c:set>
<c:set>
:向四种域设置值
- 示例
1
2
3
4
5
6
7
8
9<body>
<%--
scope:域【page, request, session, application】
var:key名
value:value值
--%>
<c:set scope="page" var="key" value="value"/>
${ pageScope.key }
</body>
2.2 <c:if>
<c:if>
:条件判断(无else)
- 示例
1
2
3
4
5
6
7
8<body>
<%--
test:判断条件(EL表达式)
--%>
<c:if test="${1 == 1}">
<span>if测试</span>
</c:if>
</body>
2.3 <c:choose><c:when><c:otherwise>
<c:choose><c:when><c:otherwise>
:多路判断(类似if … else if … else)
- 示例
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23<body>
<%--
choose:多路判断开始标签
when:多路判断(类似if / else if)
test:判断条件(EL表达式)
otherwise:末尾判断(类似else)
--%>
<c:set scope="page" var="score" value="80"/>
<c:choose>
<c:when test="${pageScope.score >= 90}">
<span>优秀</span>
</c:when>
<c:when test="${pageScope.score >= 80}">
<span>良好</span>
</c:when>
<c:when test="${pageScope.score >= 60}">
<span>及格</span>
</c:when>
<c:otherwise>
<span>不及格</span>
</c:otherwise>
</c:choose>
</body>
2.4 <c:forEach>
<c:forEach>
:用于循环遍历数据
1 | <body> |
- 补充:forEach标签还有一个额外参数,varStatus,里面存放遍历元素的信息
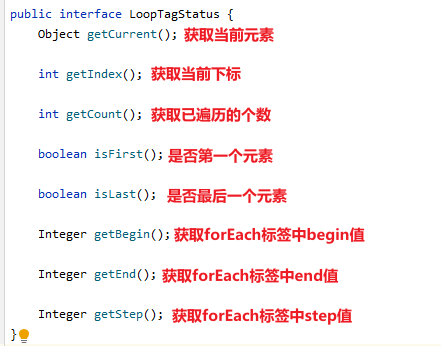
1 | <body> |