文件上传和下载实现
1 文件上传
1.1 上传
- 页面
- 使用form表单提交数据,提交方法用post
- from表单设置提交数据类型,
encType="multipart/from-data"
- 用
<input type="file">
来上传图片1
2
3
4
5
6
7<body>
<form method="post" enctype="multipart/form-data" action="http://localhost:8080/10_fileUpDown/uploadServlet">
用户:<input type="text" name="username" /> <br/>
头像:<input type="file" name="avatar" /> <br/>
<button type="submit">提交</button>
</form>
</body>
- 服务器
- 创建servlet接受数据
- mutipart/from-data传输的数据为byte流
1
2
3
4
5
6
7
8
9public class UploadServlet extends HttpServlet {
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
// multipart/form-data数据读取方式
byte[] buffer = new byte[2048000];
ServletInputStream sis = req.getInputStream();
int len = sis.read(buffer);
System.out.println(new String(buffer, 0, len));
}
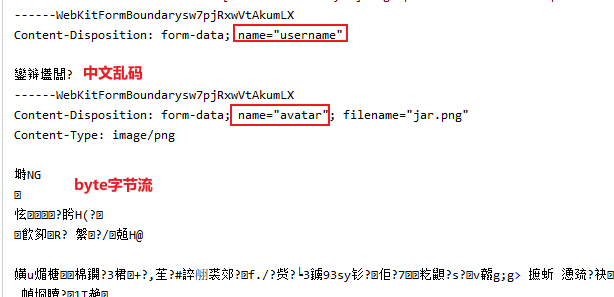
1.2 文件上传协议介绍
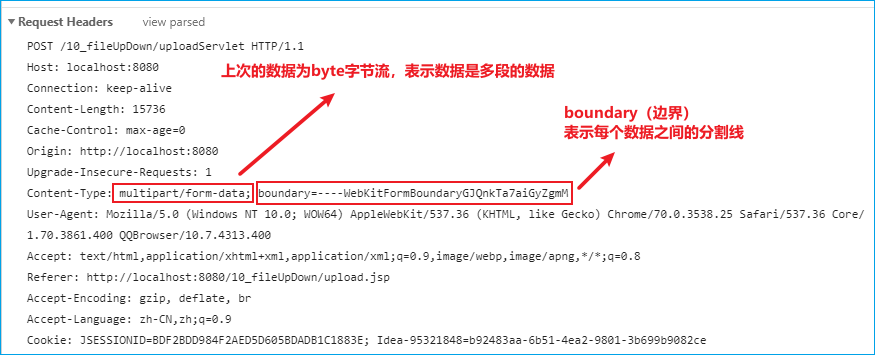
1.3 multipart数据处理
- 依赖
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20<!-- 文件上传 -->
<!-- https://mvnrepository.com/artifact/commons-fileupload/commons-fileupload -->
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.4</version>
</dependency>
<!-- https://mvnrepository.com/artifact/commons-io/commons-io -->
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.11.0</version>
</dependency>
<!-- servlet(tomcat10以下) -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>servlet-api</artifactId>
<version>2.5</version>
</dependency>
- Servlet
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27public class UploadServlet extends HttpServlet {
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
try {
// 判断是否multipart数据
RequestContext requestContext = new ServletRequestContext(req);
if (ServletFileUpload.isMultipartContent(requestContext)) {
// 解析数据
ServletFileUpload servletFileUpload = new ServletFileUpload(new DiskFileItemFactory());
List<FileItem> fileItems = servletFileUpload.parseRequest(requestContext);
// 遍历数据
for (FileItem fileItem : fileItems) {
if (fileItem.isFormField()) {
// 普通字段处理(中文注意乱码)
System.out.println(fileItem.getFieldName() + "=" + fileItem.getString("UTF-8"));
} else {
// 文件处理
System.out.println(fileItem.getFieldName() + "=" + fileItem.getName());
fileItem.write(new File("D:\\WorkSpace\\workspace-idea\\workspace01\\JavaWeb\\10_文件上传和下载\\src\\main\\resources\\" + fileItem.getName()));
}
}
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
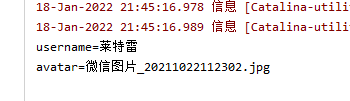
1.4 tomcat10处理
- 由于tomcat10修改了
HttpServletRequest
的包名,而commons-fileupload.jar
没对tomcat10进行适配,需要我们手动进行修改才能使用
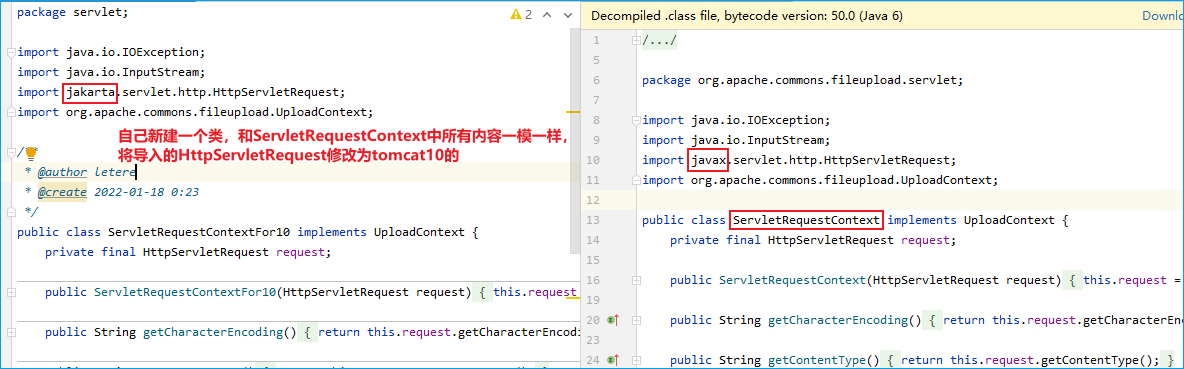
- 再将自己创建的类来构建
RequestContext
即可
2 文件下载
- 依赖
1
2
3
4
5
6
7<!-- IO工具类 -->
<!-- https://mvnrepository.com/artifact/commons-io/commons-io -->
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.11.0</version>
</dependency>
- 服务器
- 创建Servlet处理文件下载
- 下载文件需要设置response的响应类型
Content-Type
,以及数据处理方式Content-Disposition
- 读取文件,将输入流的内容移动到response中的输出流
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17public class DownloadServlet extends HttpServlet {
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
// 1 获取下载文件名
req.setCharacterEncoding("UTF-8");
String filename = req.getParameter("filename");
// 2 response设置
// 2.1 文件的类型
resp.setContentType(req.getServletContext().getMimeType("/file/" + filename));
// 2.2 数据处理方式(附件)[中文需要编码处理]
resp.setHeader("Content-Disposition", "attachment;filename=" + URLEncoder.encode(filename, "UTF-8"));
// 3 获取文件输入流,并转为输出流
// 如果文件保存在web目录下,可以通过ServletContext来获取输入流
InputStream inputStream = req.getServletContext().getResourceAsStream("/file/" + filename);
IOUtils.copy(inputStream, resp.getOutputStream());
}
}